I love using Async and Await. It makes asynchronous programming so much easier. One area I have never really liked though is the functionality for talking back to the main thread (like the UI thread) when you are doing asynchronous things though. Something I have started doing recently (and for some reason had lost from my toolbox of techniques) is the use of Delegates and Events.
In the class that has a method that runs asynchronously, I delcare some public events. These events can then be tied up on the UI thread. Then when the event is raised in the asynchronous method, the call is made back to the UI thread and it has the correct context. I haven't looked in depth into how this achieved but it works very well and gives the class with the async method on it a lot of flexbility as it's not tied to the calling class in anyway.
Eventing for VB.NET on the .NET Compact Framework
I don't know why I enjoy programming devices like this but I used to write stock management software for supermarkets using devices like this and I still sometimes have a hankering to go back to the Compact Framework and VB.NET days.
It's been a while since I did any work on the Compact Framework, but every project I come across tends to fall into foolery because of the use of Forms. Anyway, I enjoy the ability to be able to fire off events from a Form so that actions on a form have side effects, you could sort of do this when actual Events in the traditional sense but then you are tightly coupling the form and the event. Using my library below, you can publish events from the form and then both the form and then EventHandlers can be independent from one another. This means you can fire the events off from anywhere (ie a test, or a different form) and you don't need to do any wiring up.
Take a look at the code here: https://github.com/DominicFinn/Eventing
It's been a while since I did any work on the Compact Framework, but every project I come across tends to fall into foolery because of the use of Forms. Anyway, I enjoy the ability to be able to fire off events from a Form so that actions on a form have side effects, you could sort of do this when actual Events in the traditional sense but then you are tightly coupling the form and the event. Using my library below, you can publish events from the form and then both the form and then EventHandlers can be independent from one another. This means you can fire the events off from anywhere (ie a test, or a different form) and you don't need to do any wiring up.
Take a look at the code here: https://github.com/DominicFinn/Eventing
Split String Function for Excel
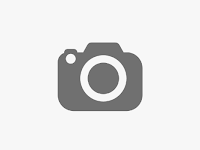
I couldn't seem to find a reasonable string splitting function in excel and I hate the foulness of using the LEFT() and FIND() function for such things.
This function below gives you the ability to split a string and return a value from it's split array.
You can use it like this....
Which will return " billy"
Really, to make it a little more robust you may want to check the length of the array created from the split and make sure that the array value you are trying to get is available given the length.
This function below gives you the ability to split a string and return a value from it's split array.
You can use it like this....
hello, billy | =SplitString(A2, ",", 1) |
Which will return " billy"
Really, to make it a little more robust you may want to check the length of the array created from the split and make sure that the array value you are trying to get is available given the length.
Generics in VB.NET
Saw these cups knocking about recently. |
I like the implementation of Generics in VB.NET. When you describe a generic type in C# or Java, and you have a type Database<T> you say a "database of T". VB.NET takes this further, the syntax is just Database(of T).
Generics allow you to resuse a class or a method for multiple types that need the same abstract functionality. A good example is two entities in your system that need to be persisted. The entities have different logic and a different reason to exist but they both need to be saved to a database. In this case you can implement the same functionality twice or reuse one class but allow it to be flexible enough to handle both entities.
I saw the picture of that cup knocking about on Twitter recently then I saw people wearing TShirts at a recent Scott Gu talk on Microsoft Azure. I would like to get my hands on an official one!
XML Literals
I love XML Literals in VB.NET. They're a great way of generating valid XML instead of dealing with giant concatenated strings.
After discussing testing an XML Processing type class with a colleague this morning I thought I'd show him how this could be achieved quite nicely using XML literals. In his case; the code base is C# but that's not a problem for us.
We are able to create an XML document directly in the code instead of concatenating a string and parsing that into an XDocument. You get really nice intellisense whilst creating the document and you can also loop over a collection for example to generate the XML.
The whole project can be found on my Github here: https://github.com/DominicFinn/XmlLiteralsForTesting
Let me know if you like it! For me, it's all part of the "write language for the job" ethos.
Beth Massi is a good source of VB.NET tips in general, this are some nice tricks that you can use XML Literals for: http://blogs.msdn.com/b/bethmassi/archive/2007/10/26/xml-literals-tips-tricks.aspx
She also did a DotNetRocks TV episode on XML Literals that you can find here: http://www.dnrtv.com/default.aspx?showNum=119
Anonymous Types
Anonymous Types are classes that you don't have to explicitly create whilst coding. They're a bit of syntactic sugar that means you can concentrate on a specific task (such as transforming and transferring data from one place to another, or filtering and totaling sets of data) instead of having to create a specific class for that one particular tasks.
For me the benefit of using them is that
For me the benefit of using them is that
- You don't have to maintain another class for a small local calculation.
- You make the code more readable, concentrating on the tasks instead of the syntax.
- You don't have a class someone can abuse for their own purposes.
The example below introduces a couple of other ideas such as Linq but we can ignore them for now. From a list of Dogs the name property alone is selected (mapped) into an anonymous object and returned as a collection.
If I recall, what the compiler nicely does for you is to create a class for you in the background that you don't see. We can check this by using the ILDASM tool that comes with Visual Studio. If you see the picture below after I load my dll into there, the anonymous type has been created as a class called AnonymousType_0`1<T0> and you can see the property Name on there as well. It's worth noticing here that the property Name is readonly meaning that the anonymous type is immutable once you have created it. Immutability is something very dear to me being a fan of F# as well as BASIC languages.
VB.NET 14 Features
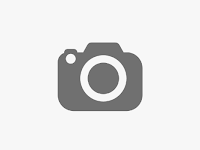
I read this article about some of the best features coming for VB.NET 14 in the next Visual Studio Release. Some of these features have been a given in C# for many years but have annoyingly been left out of VB.NET projects (The references folder for crying out loud!!!)
https://msdn.microsoft.com/en-us/magazine/dn890368.aspx
Probably my favourite of all of these additions is the ability to do Lambda expressions in a Watch window. This has been a constant source for upset for so long, if you get an enormous collection at Debug time, having to write something to iterate over them and then writing a condition or creating a conditional break point to catch what you are looking for is so so upsetting.
I knew with the Roslyn compiler C# had been rewritten from the ground up in C# (instead of C++) but I was surpsised to find out that VB.NET has also been rewritten in VB.NET and that it had resulted in a 50% speed increase. That speed increase isn't something I would expect. I am not saying VB.NET was ever slow but surely being able to run closer to the metal with C++ would result in better performance. I wonder if it's the rewrite (and therefore refactor) of ancient unwieldy code that has produced the speed increase primarily.
https://msdn.microsoft.com/en-us/magazine/dn890368.aspx
Probably my favourite of all of these additions is the ability to do Lambda expressions in a Watch window. This has been a constant source for upset for so long, if you get an enormous collection at Debug time, having to write something to iterate over them and then writing a condition or creating a conditional break point to catch what you are looking for is so so upsetting.
I knew with the Roslyn compiler C# had been rewritten from the ground up in C# (instead of C++) but I was surpsised to find out that VB.NET has also been rewritten in VB.NET and that it had resulted in a 50% speed increase. That speed increase isn't something I would expect. I am not saying VB.NET was ever slow but surely being able to run closer to the metal with C++ would result in better performance. I wonder if it's the rewrite (and therefore refactor) of ancient unwieldy code that has produced the speed increase primarily.
Subscribe to:
Posts
(
Atom
)